Tugas 2 - Pemrograman Orientasi Objek
Nama: Donny Fitrado
NRP : 05111740000171
Kelas: A
Berikut ini adalah source codenya:
1. myMain:
2. Circle:
3. Square
4. Rectangle
5. Triangle
6. Rhombus
7. Paralellogram
Berikut ini adalah gambar dari strukturnya:
NRP : 05111740000171
Kelas: A
Berikut ini adalah source codenya:
1. myMain:
import static java.lang.Math.sqrt;
import java.awt.*;
/**
* Write a description of class Main here.
*
* Donny Fitrado
* 10-9-2018
*/
public class myMain
{
public static void main(String args[])
{
//Circle
Circle aCircle;
aCircle = new Circle();
aCircle.SetRadius(3.0);
aCircle.SetCenter(2.0,2.0);
double LuasLingkaran = aCircle.Area();
double KelilingLingkaran = aCircle.Circumference();
System.out.println("Luas lingkaran adalah " + LuasLingkaran + " cm^2");
System.out.println("Keliling lingkaran adalah " + KelilingLingkaran + " cm");
//Square
Square aSquare;
aSquare = new Square();
aSquare.SetSide(4.0);
aSquare.SetCenter(2.0,2.0);
double LuasPersegi = aSquare.Area();
double KelilingPersegi = aSquare.Circumference();
System.out.println("Luas persegi adalah " + LuasPersegi + " cm^2");
System.out.println("Keliling persegi adalah " + KelilingPersegi + " cm");
//Rectangle
Rectangle aRectangle;
aRectangle = new Rectangle();
aRectangle.SetSide(4.0,3.0);
aRectangle.SetCenter(2.0,2.0);
double LuasPersegiPanjang = aRectangle.Area();
double KelilingPersegiPanjang = aRectangle.Circumference();
System.out.println("Luas persegi-panjang adalah " + LuasPersegiPanjang + " cm^2");
System.out.println("Keliling persegi-panjang adalah " + KelilingPersegiPanjang + " cm");
//Triangle
Triangle aTriangle;
aTriangle = new Triangle();
aTriangle.SetBottom(4.0);
aTriangle.SetCenter(2.0,2.0);
double LuasSegitiga = aTriangle.Area();
double KelilingSegitiga = aTriangle.Circumference();
System.out.println("Luas segitiga adalah " + LuasSegitiga + " cm^2");
System.out.println("Keliling segitiga adalah " + KelilingSegitiga + " cm");
//Rhombus
Rhombus aRhombus;
aRhombus = new Rhombus();
aRhombus.SetDiagonal(2.0,1.5);
aRhombus.SetCenter(2.0,2.0);
double LuasBelahKetupat = aRhombus.Area();
double KelilingBelahKetupat = aRhombus.Circumference();
System.out.println("Luas belah-ketupat adalah " + LuasBelahKetupat + " cm^2");
System.out.println("Keliling belah-ketupat adalah " + KelilingBelahKetupat + " cm");
//Parallelogram
Parallelogram aParallelogram;
aParallelogram = new Parallelogram();
aParallelogram.SetLongSide(4.0);
aParallelogram.SetHeight(2.0);
aParallelogram.SetHypo(3.0);
double LuasJajarGenjang = aParallelogram.Area();
double KelilingJajarGenjang = aParallelogram.Circumference();
System.out.println("Luas lingkaran adalah " + LuasJajarGenjang + " cm^2");
System.out.println("Keliling lingkaran adalah " + KelilingJajarGenjang + " cm");
}
}
2. Circle:
/**
* Write a description of class Circle here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Circle
{
private double radius;
private double centerx;
private double centery;
public Circle()
{
radius = 1;
centerx = 0;
centery = 0;
}
public void SetRadius(double r)
{
radius = r;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
return 2*3.14*radius;
}
public double Area()
{
return 3.14*radius*radius;
}
}
3. Square
/**
* Write a description of class Square here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Square
{
private double side;
private double centerx;
private double centery;
public Square()
{
side = 1;
centerx = 0;
centery = 0;
}
public void SetSide(double s)
{
side = s;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
return 4 * side;
}
public double Area()
{
return side * side;
}
}
4. Rectangle
import java.awt.*;
import java.awt.Canvas;
/**
* Write a description of class Rectangle here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Rectangle
{
private double aside;
private double bside;
private double centerx;
private double centery;
private boolean isVisible;
private String color;
public Rectangle()
{
aside = 2;
bside = 1;
centerx = 0;
centery = 0;
isVisible = true;
color = "black";
}
public void SetSide(double l, double s)
{
aside = l;
bside = s;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
return 2*aside + 2*bside;
}
public double Area()
{
return aside * bside;
}
}
5. Triangle
import static java.lang.Math.sqrt;
/**
* Write a description of class Triangle here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Triangle
{
private double bottom;
private double height;
private double hypo;
private double centerx;
private double centery;
public Triangle()
{
bottom = 1;
height = 1;
centerx = 0;
centery = 0;
}
public void SetBottom(double s)
{
bottom = s;
}
public void SetHeight(double h)
{
height = h;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
hypo = Math.sqrt(0.5*bottom*0.5*bottom + height*height);
return 2*hypo + bottom;
}
public double Area()
{
return 0.5 * bottom * height;
}
}
6. Rhombus
import static java.lang.Math.sqrt;
/**
* Write a description of class Rhombus here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Rhombus
{
private double FirstDiagonal;
private double SecondDiagonal;
private double side;
private double centerx;
private double centery;
public Rhombus()
{
FirstDiagonal = 1;
SecondDiagonal = 1;
centerx = 0;
centery = 0;
}
public void SetDiagonal(double d1, double d2)
{
FirstDiagonal = d1;
SecondDiagonal = d2;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
side = Math.sqrt(0.5 * FirstDiagonal * 0.5 * FirstDiagonal + 0.5 * SecondDiagonal * 0.5 * SecondDiagonal);
return 4 * side;
}
public double Area()
{
return 0.5 * FirstDiagonal * SecondDiagonal;
}
}
7. Paralellogram
/**
* Write a description of class Parallelogram here.
*
* Donny Fitrado
* 10-9-2018
*/
public class Parallelogram
{
private double LongSide;
private double height;
private double hypo;
private double centerx;
private double centery;
public Parallelogram()
{
LongSide = 6;
height = 4;
hypo = 5;
centerx = 0;
centery = 0;
}
public void SetLongSide(double l)
{
LongSide = l;
}
public void SetHeight(double h)
{
height = h;
}
public void SetHypo(double hy)
{
hypo = hy;
}
public void SetCenter(double x, double y)
{
centerx = x;
centery = y;
}
public double Circumference()
{
return 2 * LongSide + 2 * hypo;
}
public double Area()
{
return 0.5 * LongSide * height;
}
}
Berikut ini adalah gambar dari strukturnya:
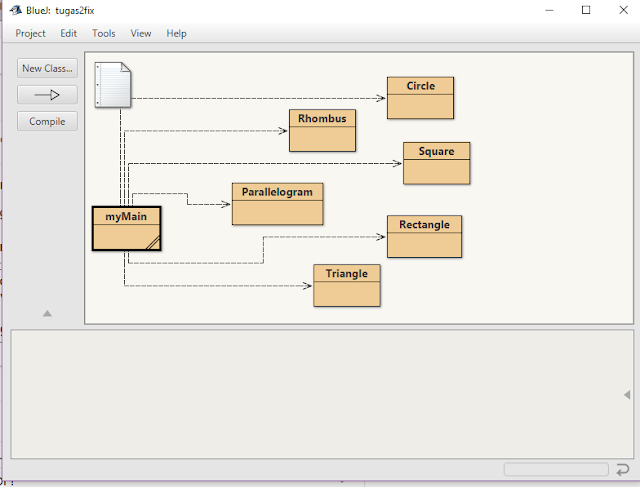
Comments
Post a Comment